异常类层次结构图-绘制变形虫结形态构图
异常一、异常简介
异常系统保证程序的健壮性
二、java异常架构
Error:与JVM相关的不可恢复的错误,如:内存溢出、JVM错误等,常见的Error
RuntimeException:运行时异常:程序运行时抛出,通过反复测试可以避免,不应该被异常处理机制解决。
运行时异常说明
算术异常
算术异常,例如被零除
ArrayIndexOutOfBoundsException异常
数组下标越界异常
空指针异常
访问空对象引用
运行时异常
运行时异常父类
数字格式异常
数字格式异常
java.lang.ClassCastException异常
类型转换异常
CheckedException: Checked exception: 编译器对代码进行检查,如果没有处理异常,则不允许程序通过。
检查异常说明
中断异常
线程中断异常
FileNotFoundException异常
找不到文件异常
异常
I/O 流异常
ClassNotFoundException异常
找不到类异常
3. 异常处理 3.1 不处理
运行时异常
3.2 捕获
try{
//可能会出现异常,需要接受监视的代码
}catch(异常类型 e){
//当异常发生后,处理预案
}
try{
//可能会出现问题,需要接受监视的代码
}catch(具体的异常类 e){
}finally{
//释放资源
}
/**
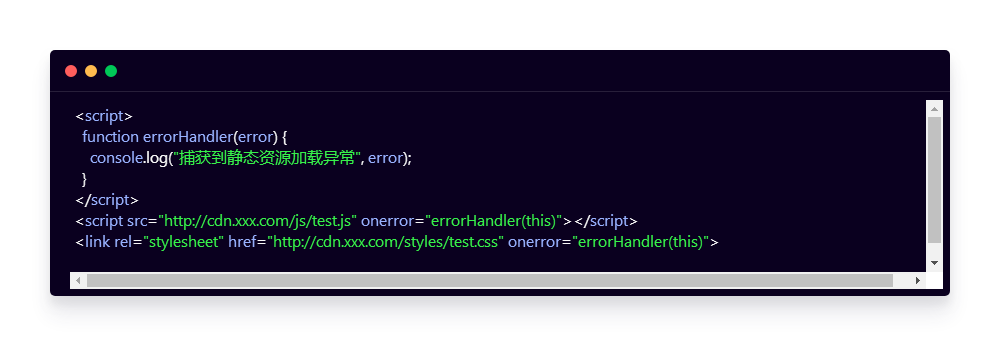
如果try 和catch块中,有return语句,finally在return代码之前执行
如果在try 和catch 块中有System.exit(0)退出虚拟机操作,则finally代码块中的语句不再执行
*/
在一个 try 块中,可能会产生多个异常,随后会产生多个 catch 来捕获不同的异常。 如果try块中出现异常,直接进入对应的catch块,try块中出现异常后的代码将不会继续执行。
try{
}catch(异常类型1 e){
}catch(异常类型2 e){
}
public class Test1 {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入两个数字");
int x = scanner.nextInt();
int y = scanner.nextInt();
int[] m = new int[10];
try {
int z = x / y;//y==0
System.out.println(z);
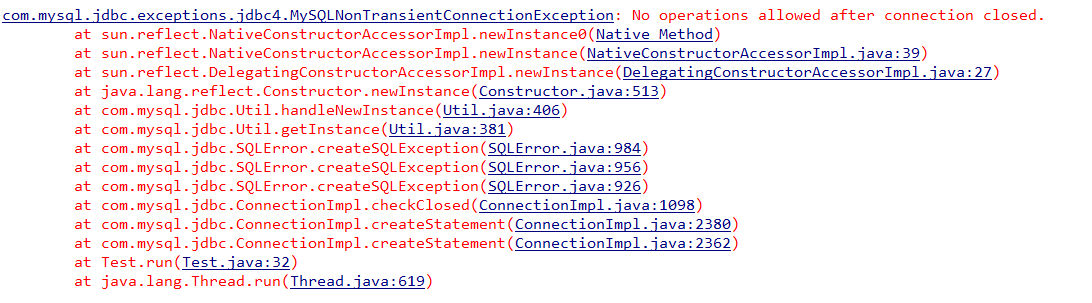
m[10] = 100;//数组下标越界
System.out.println(m[10]);
} catch (ArithmeticException e) {
System.out.println("除数不能为零");
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println("数组下标越界");
} catch (Exception e) {//必须在最后出现
System.out.println("出现错误");
}
System.out.println("");
}
}
JDK7版本以后,一个catch块可以捕获多个异常
Scanner scanner = new Scanner(System.in);
System.out.println("请输入两个数字");
int x = scanner.nextInt();
int y = scanner.nextInt();
int[] m = new int[10];
try {
int z = x / y;//y==0
System.out.println(z);
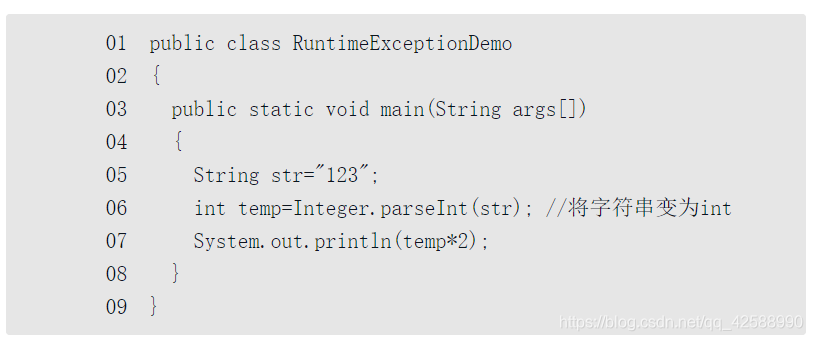
m[10] = 100;//数组下标越界
System.out.println(m[10]);
}catch (ArithmeticException | ArrayIndexOutOfBoundsException e){//>=JDK7版本
//获取错误消息
System.out.println("出现错误:"+e.getMessage());
}
处理异常消息
如果try和catch操作是嵌套的,如果内层catch不能处理内层try代码块的异常java异常类层次结构图,就会被外层catch块捕获
3.3 投掷
抛出,抛出关键字
抛出用法:
确定问题,反馈给来电者,由来电者处理
方法中使用
完全异常抛出,如果某个方法可能发生异常,当前方法不处理,抛到方法异常列表中,使用throws关键字。 可以接收多个异常,用逗号分隔。
谁将调用此方法java异常类层次结构图,谁将处理异常
public static Date getDateByString(String str) throws ParseException {
DateFormat format = new SimpleDateFormat("yyyy-MM-dd");
Date parse = format.parse(str);
return parse;
}
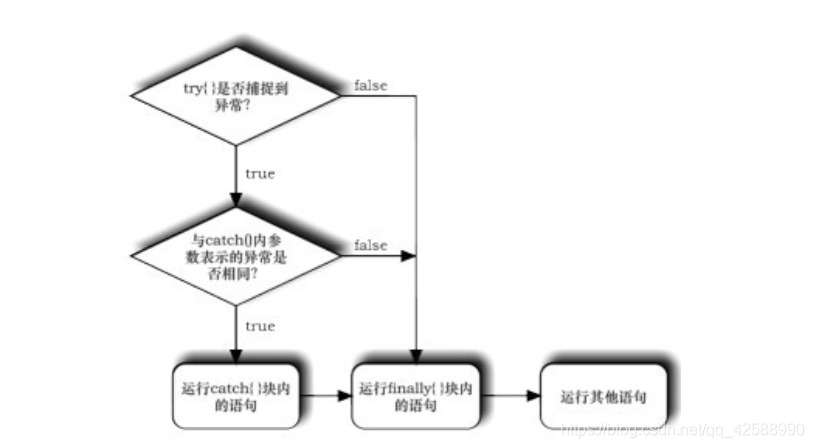
抛出用法:
使用throw 抛出指定类型的异常处理。
在方法内部使用
语法格式 throw Throwable类或其子类对象在方法体中的throw语句,如果抛出的是运行时异常,则不需要做任何处理。如果抛出的是checked异常,则不能单独使用,必须使用用 try catch 或 throws
如果在方法中使用throw抛出异常,则认为该方法执行完毕,后面不能再有语句,除非用try和catch处理异常。
public static int divide(int a, int b) {
if (b == 0) {
throw new ArithmeticException("除数不能为0");
}
return a / b;
}
3.4 自定义异常
继承Throwable及其子类来写
public class AgeException extends Exception{
public AgeException() {
super("年龄不符合条件");
}
public AgeException(String message) {
super(message);
}
}
1、什么时候用throw、throws、try...catch 2、Exception和RuntimeException的区别 3、异常对象的常用方法: